File Structure
Updated on Jul 11, 2024
After installing Laravel, it is a good idea to familiarize yourself with its file structure. Let's look at the application's root directory. You should see these directories and files.
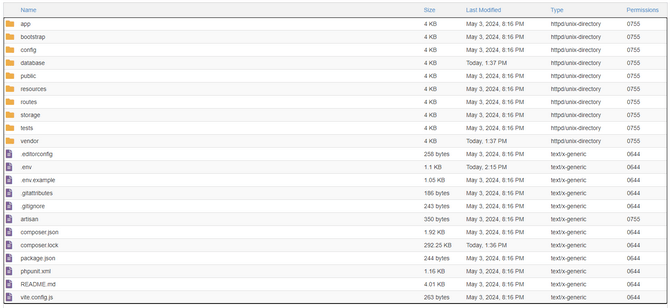
The directories are the most important thing here, as they contain the majority of the code the application needs to function. Here is a quick breakdown of each of them.
- app - This is where the base code for your Laravel application is located. This is where it will be developed;
- bootstrap - Contains all the bootstrapping scripts used for your application. The app.php file is located here, and it is the main script responsible for the bootstrapping process;
- cache - Holds cache files for your application;
- config - All your project configuration files are located here. It's a good idea to read through all of these files and familiarize yourself with the options available.
- database - The database directory contains your database files: database migrations, model factories, and seeds. If you wish, you may also use this directory to hold an SQLite database;
- public - The public directory helps start your Laravel project and maintains other necessary files such as JavaScript, CSS, and images of your project. It also has the index.php file, which is the entry point for all requests entering your application and configures autoloading;
- resources - The resources directory contains your views as well as your raw, un-compiled assets such as CSS or JavaScript;
- routes - The routes directory contains all your definition files for routing, such as web.php, console.php, api.php, channels.php, etc. Since these files are essential, let's elaborate on them.
- The web.php file contains routes the RouteServiceProvider places in the web middleware group, providing session state, CSRF protection, and cookie encryption. If your application does not offer a stateless, RESTful API, then all your routes will most likely be defined in the web.php file;
- The api.php file contains routes the RouteServiceProvider places in the API middleware group. These routes are intended to be stateless, so requests entering the application through these routes will be authenticated via tokens and will not have access to the session state;
- The console.php file is where you may define all closure-based console commands. Each closure is bound to a command instance, allowing a simple approach to interacting with each command's IO methods. Though this file does not define HTTP routes, it defines console-based entry points (routes) into your application;
- The channels.php file is where you may register all of the event broadcasting channels that your application supports.
- storage - The storage directory holds your session files, logs, cache, compiled templates, and miscellaneous files generated by the framework. This directory contains several others: app, framework, and logs. The app directory may be used to store any files generated by your application. The framework directory is used to store framework-generated files and caches. Finally, the logs directory contains your application's application's log files;
- test - The directory holds all your test cases. For example, PHPUnit and feature tests are provided out of the box. Each test class should be suffixed with the word Test. You may run your tests using the php vendor/bin/phpunit command;
- vendor - The vendor directory has all Composer dependency files.
As we mentioned, the app directory is very important, as that is where your application's code is. As you can imagine, the majority of your application is housed there, so we want to take a closer look at it and explain the different directories that can exist in it.
- Broadcasting - Contains all of the broadcast channel classes for your application. These classes are generated using the make:channel command. This directory does not exist by default but will be created when you create your first channel. If you are uncertain what channels are, you can check out Laravel's documentation;
- Console - All of the custom Artisan commands for your application are contained here. These commands may be generated using the make:command command. The directory also houses your console kernel, which is where your custom Artisan commands are registered and your scheduled tasks are defined;
- Events - Does not exist by default but will be created for you by the event:generate and make:event Artisan commands. The Events directory houses event classes. Events may be used to alert other parts of your application that a given action has occurred, providing a great deal of flexibility and decoupling;
- Exceptions - Your application's exception handler is located here and is also the place for any exceptions thrown by your application. If you would like to customize how your exceptions are logged or rendered, you should modify the Handler class in this directory;
- HTTP - Contains your controllers, middleware, and form requests. Almost all of the logic to handle requests entering your application will be placed in this directory;
- Jobs - This directory does not exist by default but will be created if you execute the make:job Artisan command. The Jobs directory houses the queueable jobs for your application. Jobs may be queued by your application or run synchronously within the current request lifecycle. Jobs that run synchronously during the current request are sometimes referred to as "commands" since they are an implementation of the command pattern;
- Listeners - This directory also does not exist by default. It will be created for you if you execute the event:generate or make:listener Artisan commands. The Listeners directory contains the classes that handle your events. Event listeners receive an event instance and perform logic in response to the event being fired. For example, a UserRegistered event might be handled by a SendWelcomeEmail listener;
- Mail - Another directory that does not exist by default. You can create it by executing the make:mail Artisan command. The Mail directory contains all of your classes that represent emails sent by your application. Mail objects allow you to encapsulate all of the logic of building an email in a single, simple class that may be sent using the Mail::send method;
- Models - Contains all of your Eloquent model classes. In a previous part of our tutorial, we talked about the Eloquent ORM included with Laravel. Each database table has a corresponding Model used to interact with that table. Models allow you to query for data in your tables, as well as insert new records into the table;
- Notifications - You can create this directory by executing the make:notification Artisan command. The Notifications directory contains all of the "transactional" notifications sent by your application, such as simple notifications about events within your application. Laravel's notification feature abstracts sending notifications over a variety of drivers such as email, Slack, SMS, or stored in a database;
- Policies - Create the directory by executing the make:policy Artisan command. The Policies directory contains the authorization policy classes for your application. Policies are used to determine if a user can perform a given action against a resource;
- Providers - Contains all of the service providers for your application. Service providers bootstrap your application by binding services in the service container, registering events, or performing any other tasks to prepare your application for incoming requests. We explained them earlier in this tutorial as well. You are free to add your own providers to this directory as needed, in addition to the ones that come with Laravel by default;
- Rules - Execute the make:rule Artisan command to create this directory. It contains the custom validation rule objects for your application. Rules are used to encapsulate complicated validation logic in a simple object.
As you can see, many directories are not created by default and need to be created either manually or by executing a command. That is good if you want to keep your directory structure tidy in case your application does not use those specific functions. That is why we said the app directory is vital to your application. The vast majority of its functionality will be programmed in it.
This concludes our look at the Laravel directory structure. It is tidy and straightforward, and we hope our descriptions will help you understand it better.